Variable Storage
The Variable Storage is responsible for storing the values of Yarn variables (see Expressions and Variables).
Yarn Spinner is designed to work alongside an existing data storage system, so ideally you customize your own variable storage, or integrate with your chosen third-party solution.
InMemoryVariableStorage
Yarn Spinner comes with a very simple in-memory variable storage system, which keeps everything in memory and doesn’t automatically save anything to disk by default. If you want to write your own variable storage system, it can be a useful reference.
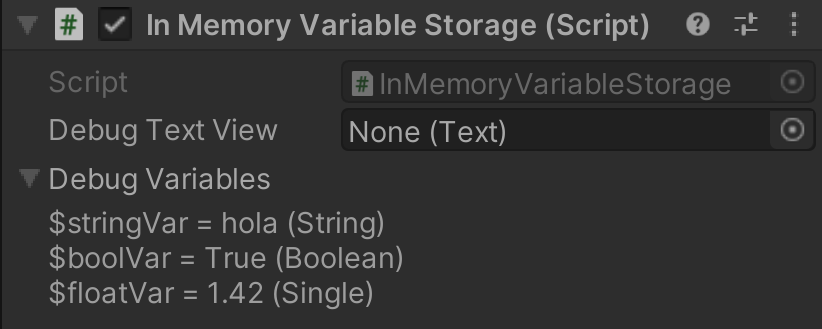
- Debug Text View: Optional. Lists all variables and current values with a Unity UI Text. Good for debug builds.
- Debug Variables: Lists all variables and current values, but in the inspector itself. When debugging in editor, this might be faster or more convenient than Debug Text View.
Saving / loading / serializing variable data
Serialization is the process of converting program state into data, while deserialization is the process of reconstructing program state from data. In games, we often use serialization for “save file” functionality.
As of v2.0, the default InMemoryVariableStorage
stores variable types (bool
, string
, float
) as System.Object
types in a dictionary. This can be a bit tricky to serialize, so as an example we’ve implemented some basic serialization functions like SerializeAllVariablesToJSON()
and DeserializeAllVariablesFromJSON()
using Unity’s built-in JSONUtility. Some example JSON data:
{
"keys":[
"System.String/$stringVar",
"System.Boolean/$boolVar",
"System.Single/$floatVar"
],
"values":[
"hola",
"True",
"1.42"
]
}
We also have two example save / load implementations that wrap around the serialization functions: LoadFromFile()
and SaveToFile()
read / write .json files (we recommend using Unity’s Application.persistentDataPath
as part of the filepath), while LoadFromPlayerPrefs()
and SaveToPlayerPrefs()
use Unity’s built-in PlayerPrefs system.
Create a variable storage from scratch
To create your own variable storage from scratch, implement the VariableStorageBehaviour
abstract class, itself a subclass of MonoBehaviour
.
-
Create a new C# script, and call it (for example)
CustomVariableStorage.cs
. -
Next, change the parent class from
MonoBehaviour
toYarn.Unity.VariableStorageBehaviour
. -
Finally, add the following methods to it. You will need to add your own behaviour:
public class CustomVariableStorage : Yarn.Unity.VariableStorageBehaviour {
// main function used by Dialogue Runner to retrieve Yarn variable values
public override bool TryGetValue<T>(string variableName, out T result) {
// retrieve value with key "variableName" from a list or dictionary, etc.
}
// overload for setting a String variable
public override void SetValue(string variableName, string stringValue) {
// save "stringValue" under key "variableName" to a dictionary, etc.
}
// overload for setting a Float variable
public override void SetValue(string variableName, float floatValue) {
// save to a dictionary, etc.
}
// overload for setting a Boolean variable
public override void SetValue(string variableName, bool boolValue) {
// save to a dictionary, etc.
}
// clear all variable data
public override void Clear() {
// clear all your dictionaries
}
}
- You’re now able to add this script to your Dialogue Runner object. Don’t forget to set the Dialogue Runner’s Variable Storage field to the component that you added.